Embedded Vulnerabilities
To demonstrate EIV’s detection and prevention abilities, several vulnerabilities are embedded in the demo project’s code. These vulnerabilities can be triggered remotely using the attack utility python script provided in the project (described in the Operations section).
A. Stack Overflow
Overview
Stack overflow is a memory corruption vulnerability affecting the stack memory. A crafted stack buffer overflow exploitation can lead to arbitrary code execution, for example, by overflowing a stack buffer and corrupting the return address which is saved on the stack. Thus, when the vulnerable function ends, it will return to a malicious address that will contain some malicious code, which will be executed on the device (instead of the original code in the original return address). Thus, for full exploitation, both the memory and the flow integrity will be violated. The following is an example of a function vulnerable to a stack overflow:
void stack_overflow(char *s)
{
char buffer[4];
strcpy(buffer, s);
}
Invoking the stack_overflow() function with a string that is longer than 4 characters will exceed the boundaries of the buffer stack allocation and corrupt the stack as described below:
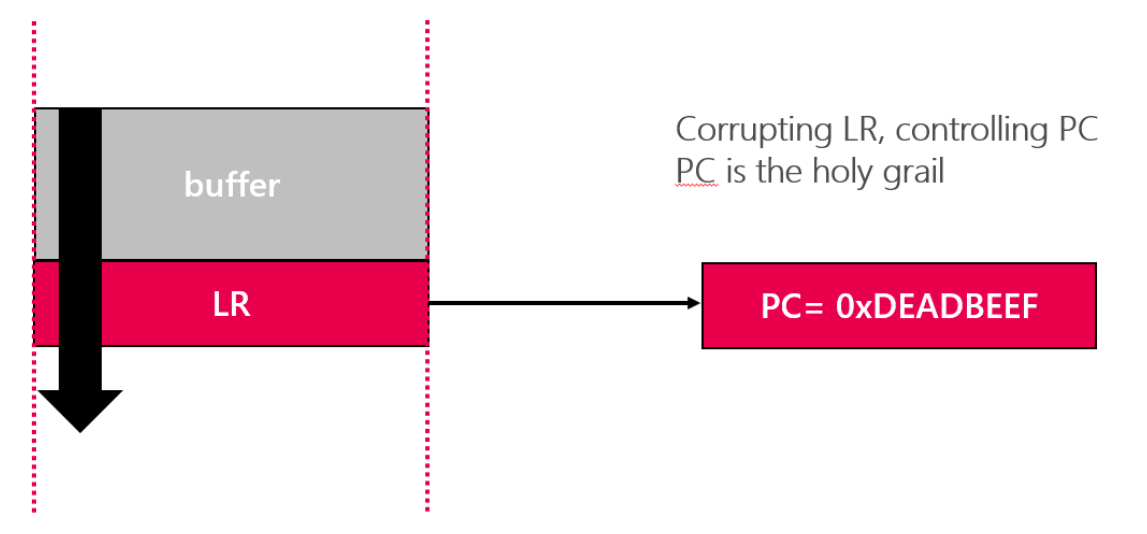
In ARM:
- Function return address is saved in the Link Register (LR) when using BL or BLX.
- PC is the register that holds the address of the next opcode to be executed by the CPU.
- Functions return via restoring the PC from LR.
The “LR” register, which is responsible for the return address in ARM-based architecture, will be corrupted by the threat actors’ malicious address. Therefore, when the function exits, the CPU will start executing code from the address at LR, which now contains a malicious, controlled-by-the-hacker address.
Embedded Vulnerability
The Stack Overflow vulnerability embedded in the the demo firmware is:
volatile void printPayload_StackOverflow(MQTTPublishInfo_t * pPublishInfo)
{
volatile char infoMessage[22];
b64_decode(pPublishInfo->pPayload, packetBuffer, 300);
int messageLength = (unsigned int)(packetBuffer[0]);
packetBuffer[messageLength] = 0;
memcpy(infoMessage, packetBuffer + 1, messageLength + 1);
LogInfo( ( "Received info message: %s", infoMessage ) );
}
Triggering the Stack Overflow Vulnerability Attack
To trigger this vulnerability, run the attack_utility.py python script as follow (replace 2097775479 with your device ID):
python3 attack_utility.py -d 2097775479 -s stack_overflow
Exploitation Attempt Alert on the Sternum Platform
The exploitation attempt investigation on the Sternum Platform shows information about the vulnerable code that was attempted to be exploited during the attack.
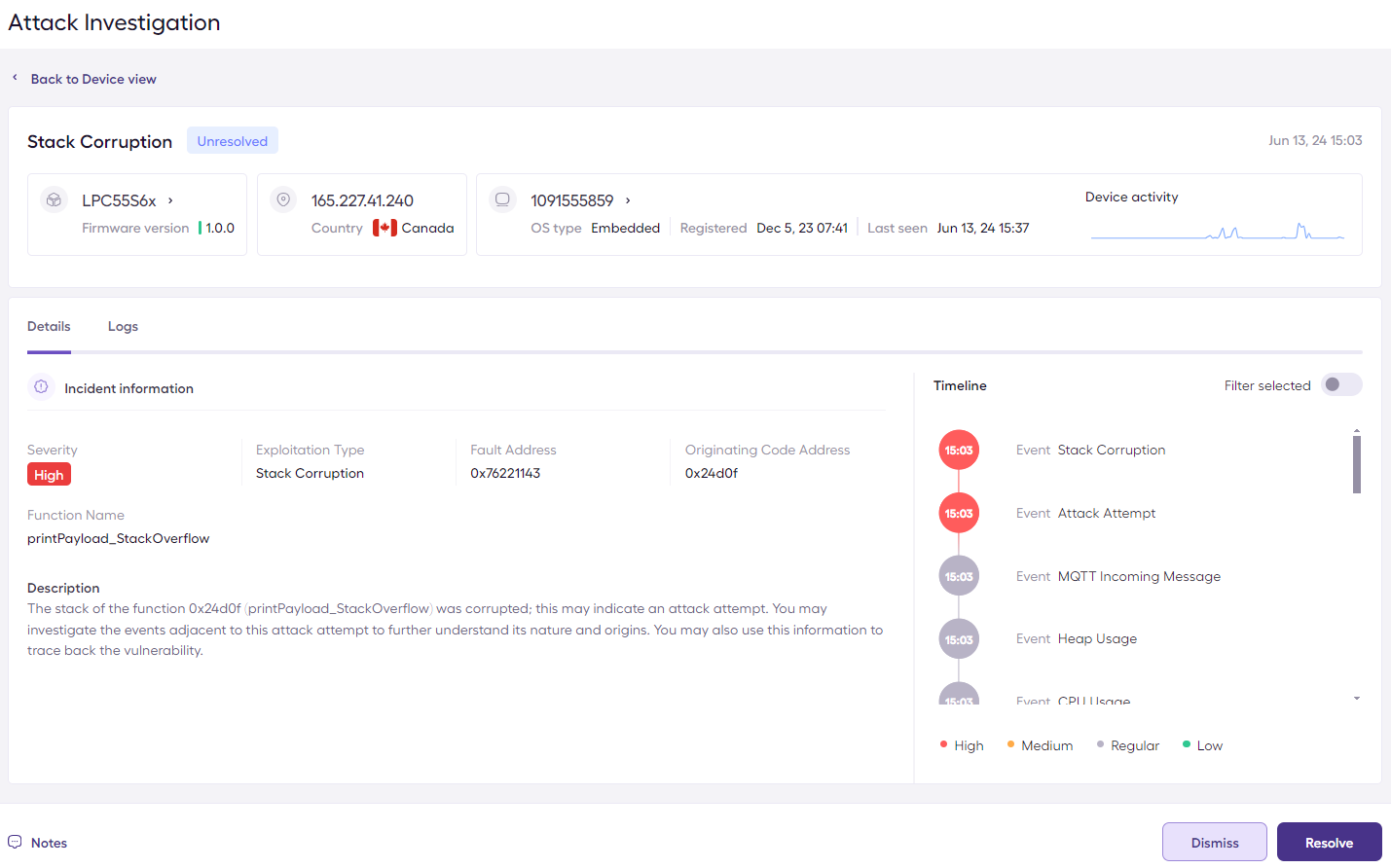
In this case, the attack originated the printPayload_StackOverflow function where the vulnerable code resides. We can also see that the vulnerability already occured right after an MQTT incoming message event.
B. Heap Overflow
Overview
Heap overflow is a memory corruption vulnerability affecting the Heap. Below you can see an illustration of a heap overflow exploitation that will eventually overflow a function pointer. When the program’s code tries to invoke the original function pointer, the malicious pointer value will be executed instead.
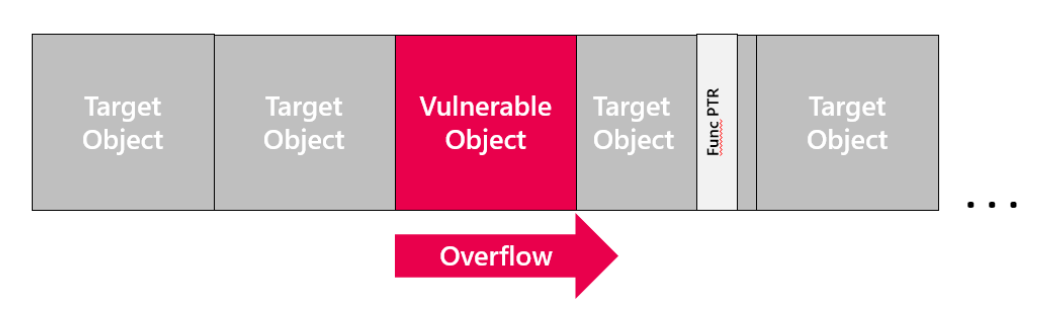
Embedded Vulnerability
The Heap Overflow vulnerability embedded in the demo firmware is:
volatile void printPayload_HeapOverflow(MQTTPublishInfo_t * pPublishInfo)
{
b64_decode(pPublishInfo->pPayload, packetBuffer, 300);
int messageLength = (unsigned int)(packetBuffer[0]);
char* buf = (char*)malloc(52);
volatile p_printer_func * pp_printer = (p_printer_func *)malloc(4);
*pp_printer = (p_printer_func)&log_string;
memcpy(buf, packetBuffer + 1, messageLength + 1);
(*pp_printer)(buf);
free(buf);
}
Triggering the Heap Overflow vulnerability attack
To trigger this vulnerability, run the attack_utility.py python script as follow (replace 2097775479 with your device ID):
python3 attack_utility.py -d 2097775479 -s heap_overflow
Exploitation Attempt Alert on the Sternum Platform
The exploitation attempt investigation on the Sternum Platform shows information about the vulnerable code that was attempted to be exploited during the attack.
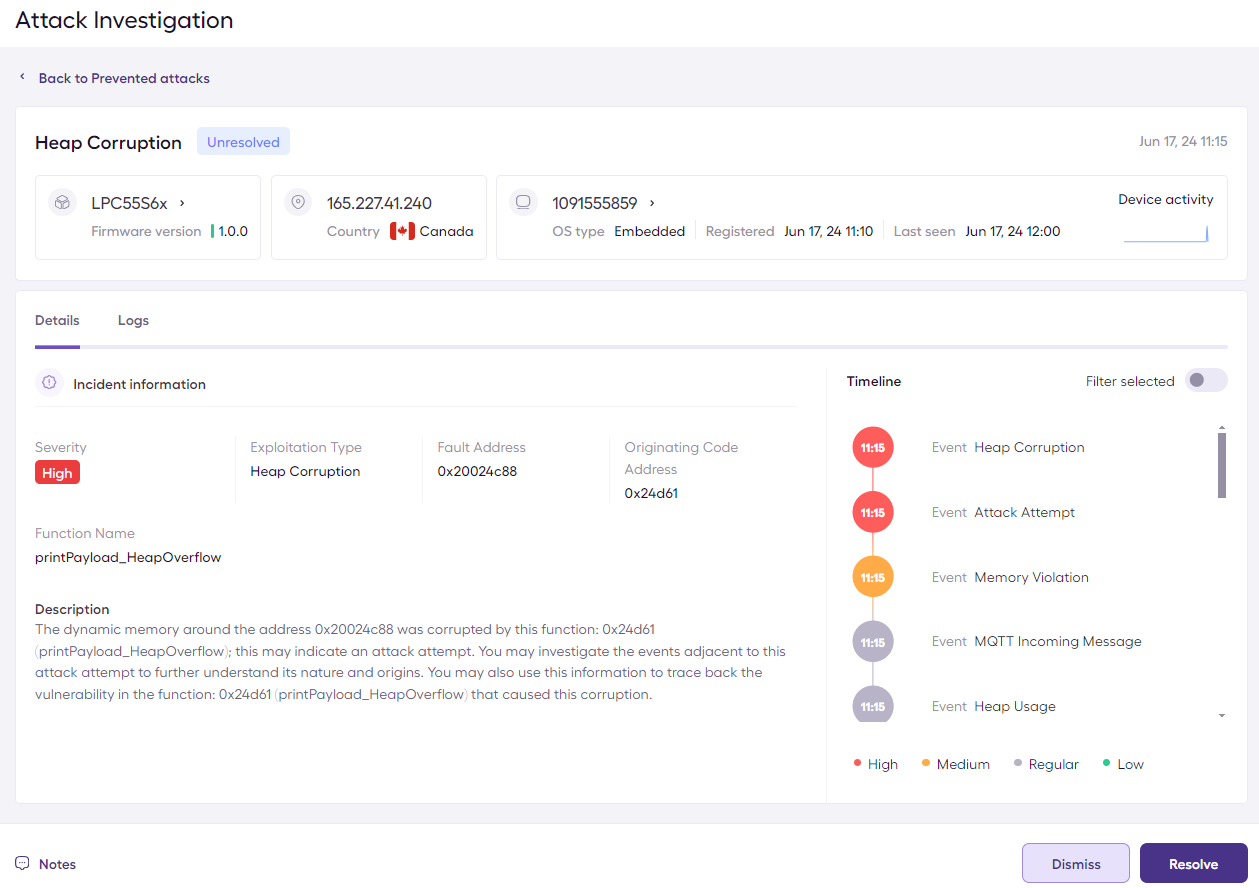
In this case, the attack originated from the printPayload_HeapOverflow function where the vulnerable code resides. We can see that the destination allocation size was 52, and a copy of 118 bytes would have taken place and corrupt the memory. We can also see that the vulnerability already occurred right after an MQTT incoming message event.
C. Use After Free
Overview
Use after free vulnerability is the usage of memory after it has been freed. Below you can see an illustration of a use after free exploitation that will replace a function pointer. When the program’s code tries to invoke the original function pointer, the malicious pointer value will be executed instead.
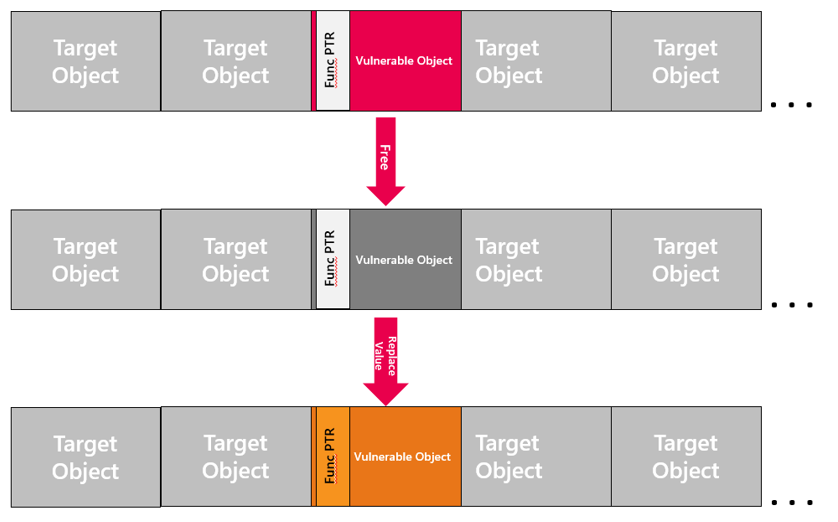
Embedded Vulnerability
The Use After Free vulnerability embedded in the demo firmware is:
volatile void printPayload_UseAfterFree(MQTTPublishInfo_t * pPublishInfo)
{
b64_decode(pPublishInfo->pPayload, packetBuffer, 300);
int messageLength = (unsigned int)(packetBuffer[0]);
char* buf = (char*)malloc(52);
volatile p_printer_func * pp_printer = (p_printer_func *)malloc(4);
memset(buf, 0, 52);
*pp_printer = (p_printer_func)&log_string;
free(buf);
free(pp_printer);
char* dateMsg = (char*)malloc(120);
memcpy(dateMsg, packetBuffer + 1, messageLength + 1);
// USE AFTER FREE
(*pp_printer)(buf);
}
Triggering the Use After Free vulnerability Attack
To trigger this vulnerability, run the attack_utility.py python script as follow (replace 2097775479 with your device ID):
python3 attack_utility.py -d 2097775479 -s use_after_free
Exploitation Attempt Alert on the Sternum Platform
The exploitation attempt investigation on the Sternum Platform shows information about the vulnerable code that was attempted to be exploited during the attack.
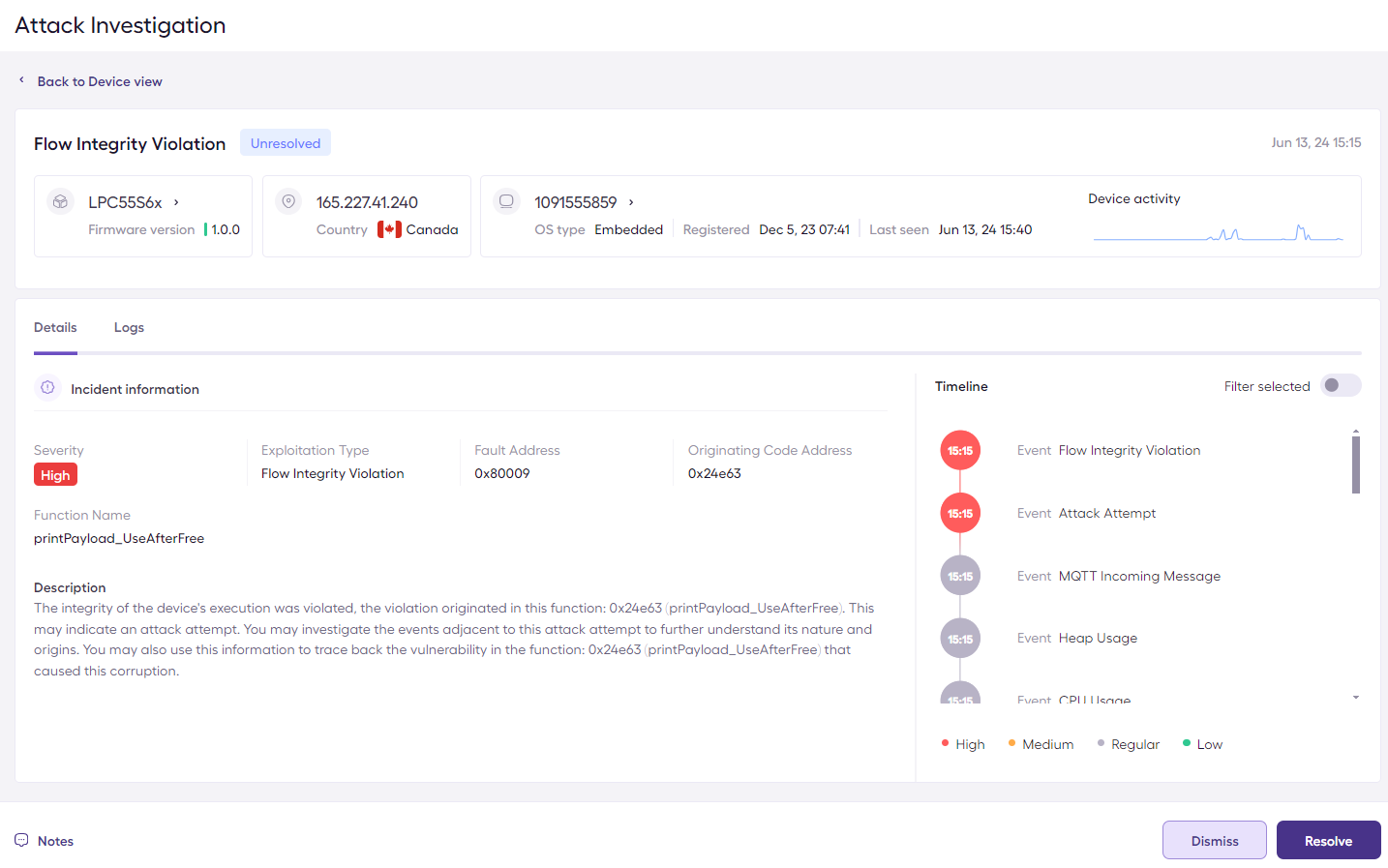
D. Control Flow violation
Overview
Control flow integrity (CFI) is a security mechanism that prevents modification of the firmware's flow of execution.
Examples of control flow attack techniques are Return-Oriented-Programming (ROP) and Jump-Oriented-Programming (JOP). Jump-Oriented Programming (JOP) is similar to Return-Oriented Programming (ROP). ROP attacks look for sequences that end in a function return. In contrast, JOP attacks target sequences that end in other forms of indirect branches, like function pointers or case statements.
Embedded Vulnerability
The flow integrity vulnerability embedded in the demo firmware is:
volatile void printPayload_FlowIntegrityViolation(MQTTPublishInfo_t * pPublishInfo)
{
b64_decode(pPublishInfo->pPayload, packetBuffer, 300);
int messageLength = (unsigned int)(packetBuffer[0]);
volatile p_printer_func pp_printer = 0;
char buf[32] = {0};
pp_printer = (p_printer_func)&log_string;
memcpy(buf, packetBuffer + 1, messageLength + 1);
(*pp_printer)(buf);
}
Triggering the Flow Integrity Vulnerability Attack
To trigger this vulnerability, run the attack_utility.py python script as follow (replace 2097775479 with your device ID):
python3 attack_utility.py -d 2097775479 -s flow_integrity_violation
Exploitation Attempt Alert on the Sternum Platform
The exploitation attempt investigation on the Sternum Platform shows information about the vulnerable code that was attempted to be exploited during the attack.
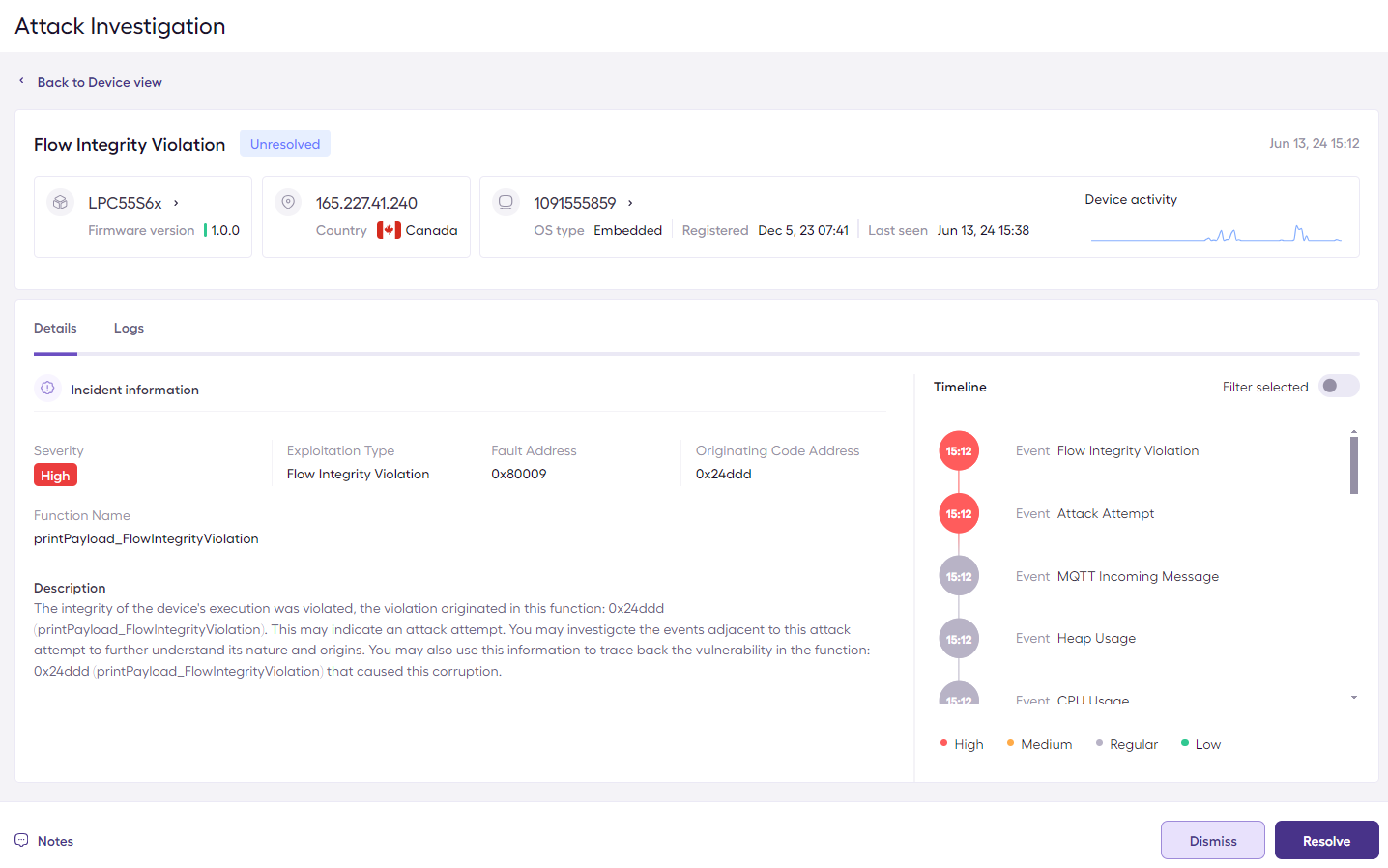